Everything You Need To Know About Arrays in PHP
Posted by TotalDC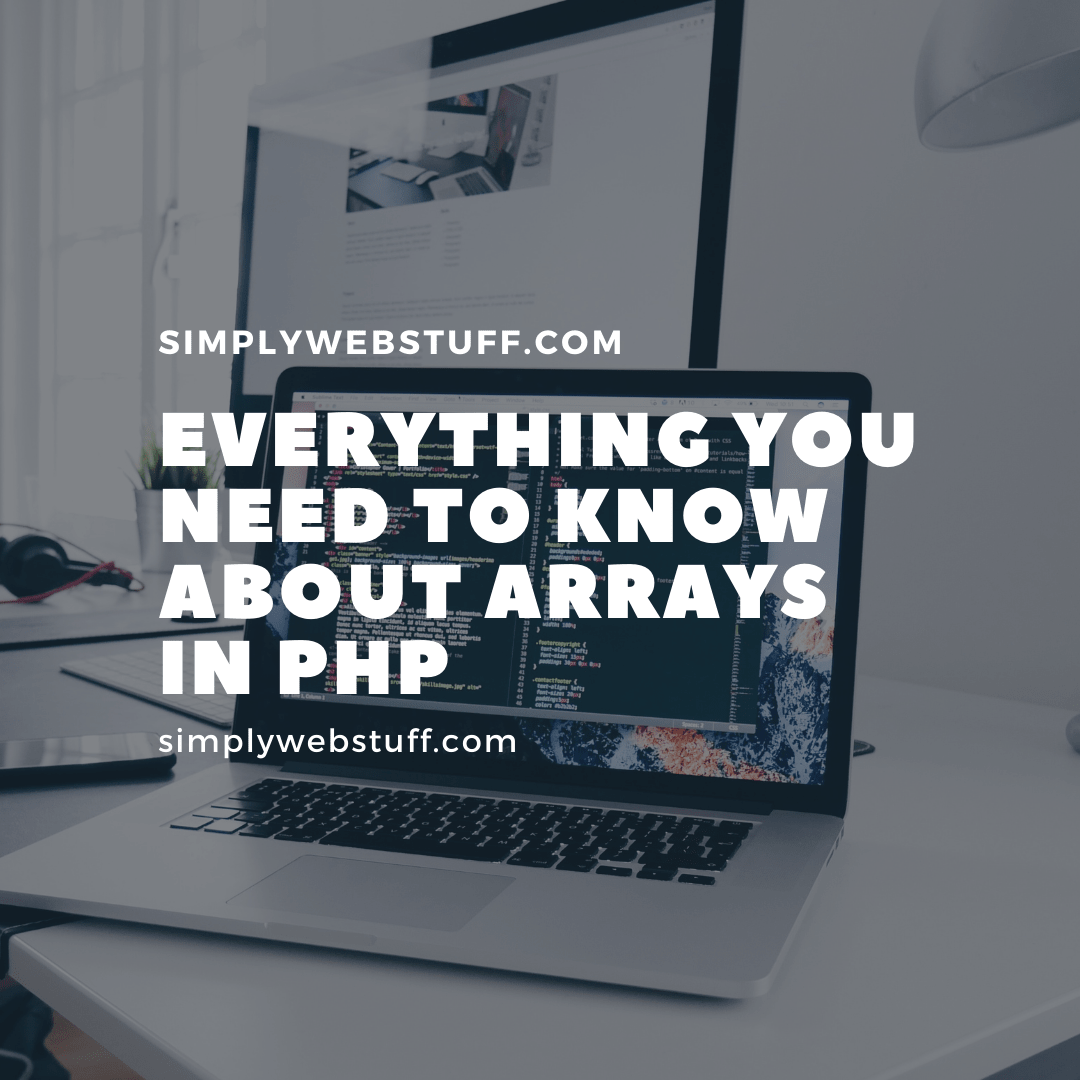
Now that we have covered such things as operators, strings, if – else and switch statements, let’s dive deeper and talk about PHP arrays.
What Is An Array In PHP
Arrays in PHP like arrays in JavaScript are variables that let you store a group of values in a single variable. Let’s say that you want to store colors. Storing colors in a different variable could look something like this:
<?php
$color1 = "Red";
$color2 = "Green";
$color3 = "Blue";
?>
But what if you want or need to store for example city names of a country, so this time you will have more than a couple of values. It is quite hard and boring to store each city name in a separate variable. And here is where you want to use the array.
Types Of Arrays in PHP
There are three types of arrays you can create:
- Indexed array — An array with a numeric key.
- Associative array — An array where each key has its specific value.
- Multidimensional array — An array containing one or more arrays within itself.
Indexed Arrays In PHP
An indexed or numeric array stores each array element with a numeric index. The following examples show two ways in which you can create an indexed array. The first and easiest way would be:
<?php
$colors = array("Red", "Green", "Blue");
?>
Or you can assign indexes manually:
<?php
$colors[0] = "Red";
$colors[1] = "Green";
$colors[2] = "Blue";
?>
Associative Arrays In PHP
In an associative array, keys are assigned to values. They can be arbitrary and user-defined strings. Here are a couple of examples where keys are used instead of index numbers:
<?php
$ages = array("Paul"=>22, "Peter"=>32, "John"=>28);
?>
Another way to create an associative array in PHP is:
<?php
$ages["Paul"] = "22";
$ages["Peter"] = "32";
$ages["John"] = "28";
?>
Multidimensional Arrays In PHP
As you may be thinking, a multidimensional array in PHP is an array in which each element can be an array, and so on. An example of a multidimensional array would look something like this:
<?php
$contacts = array(
array(
"name" => "Paul Pauler",
"email" => "paulpauler@mail.com",
),
array(
"name" => "Clark Clarkson",
"email" => "clarkclarkson@mail.com",
),
array(
"name" => "Harry Haryson",
"email" => "harryharyson@mail.com",
)
);
print "Paul Pauler's Email-id is: " . $contacts[0]["email"];
?>
Accessing Array Structure And Values In PHP
You can see the structure and values of an array by using var_dump(). Here is an example for you:
<?php
$cities = array("London", "Mexico", "New York");
var_dump($cities);
?>
This var_dump() statement gives the following result:
array(3) { [0]=> string(6) "London" [1]=> string(5) "Mexico" [2]=> string(8) "New York" }
This output shows the data type of each element, such as a string of 6 characters, in addition to the key and value.