Everything You Need To Know About JavaScript Functions
Posted by TotalDC
A JavaScript function – a set of statements that take inputs, do some specific things, and produce output. A function is a set of statements that performs some tasks and then returns the result to the user.
The idea is to put some repeatedly done tasks together and make a function so that you don’t have to write the same code again and again for different inputs.
Like other programming languages, JavaScript supports the use of functions. You probably have seen some commonly used functions in JavaScript like alert(), which is a built-in function in JavaScript. But JavaScript allows you to create user-defined functions also.
In this article you will learn:
- How To Define And Call JavaScript Function
- How To Add Parameters To A JavaScript Function
- Default Values For JavaScript Function Parameters
- How To Return Values From A JavaScript Function
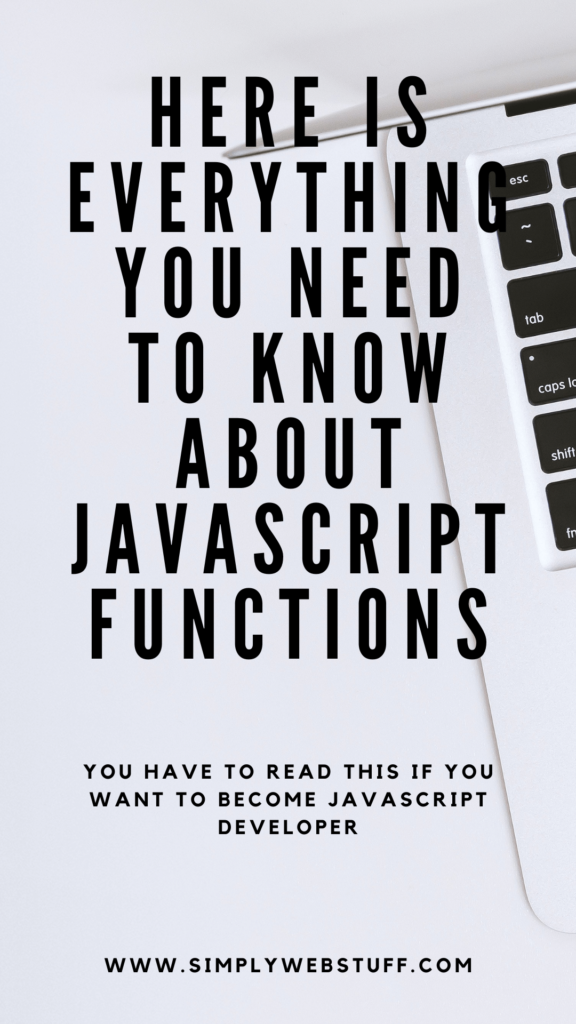
How To Define And Call JavaScript Function
The declaration of a function starts with the function keyword and then the name of the function you are creating followed by parentheses and finally – curly brackets between which you will write your function’s code. Here’s the basic syntax for declaring a function:
function functionName() {
// Code to be executed
}
Here is a simple example of a function that will give you pop up message with hello world text:
// Defining function
function sayHello() {
alert("Hello world!");
}
// Calling function
sayHello(); // Result: Hello world!
Once a function is defined it can be called (invoked) from anywhere in the document, by typing its name followed by a set of parentheses, like sayHello() in the example above.
How To Add Parameters To A JavaScript Function
You can specify parameters when you define your function to accept input values when the function is run. The parameters work like placeholder variables within a function, they are replaced at run time by the values provided to the function when the function is called.
Parameters are set on the first line of the function inside the set parentheses. Just like this:
function functionName(parameter1, parameter2, parameter3) {
// Code to be executed
}
Here’s an example of a function called displaySum() that takes two numbers as arguments and just simply adds them together and then returns the result.
// Defining function
function displaySum(num1, num2) {
let total = num1 + num2;
console.log(total);
}
// Calling function
displaySum(6, 20); // Result: 26
displaySum(-5, 17); // Result: 12
Keep in mind that you can define as many parameters as you like, but for each parameter, you have to provide a corresponding argument when a function is called if you don’t do that, you will get undefined as a result. Here’s an example:
// Defining function
function showFullname(firstName, lastName) {
console.log(firstName + " " + lastName);
}
// Calling function
showFullname("Total", "DC"); // 0utputs: Total DC
showFullname("Paul"); // 0utputs: Paul undefined
Default Values For JavaScript Function Parameters
Since ES6 you can specify default values to the function parameters. This means that if no arguments are provided to the function when it’s called the value of these parameters will be used. Here’s how it looks:
function sayHello(name = 'Guest') {
console.log('Hello, ' + name);
}
sayHello(); // 0utputs: Hello, Guest
sayHello('Paul'); // 0utputs: Hello, Paul
How To Return JavaScript Function Values
A function can return a value using the return statement. The value may be of any type, including arrays and objects.
The return statement is usually placed as the last line of the function before the closing curly bracket as shown in the example:
// Defining function
function getSum(num1, num2) {
let total = num1 + num2;
return total;
}
// Displaying returned value
console.log(getSum(6, 20)); // Result: 26
console.log(getSum(-5, 17)); // Result: 12
A function cannot return multiple values. But, you can get similar results by returning an array of values, as demonstrated in the following example.
// Defining function
function divideNumbers(dividend, divisor){
let quotient = dividend / divisor;
let arr = [dividend, divisor, quotient];
return arr;
}
// Store returned value in a variable
let all = divideNumbers(10, 2);
// Displaying individual values
console.log(all[0]); // Result: 10
console.log(all[1]); // Result: 2
console.log(all[2]); // Result: 5