JavaScript Cheat Sheet That Will Make You A Better Developer
Posted by TotalDC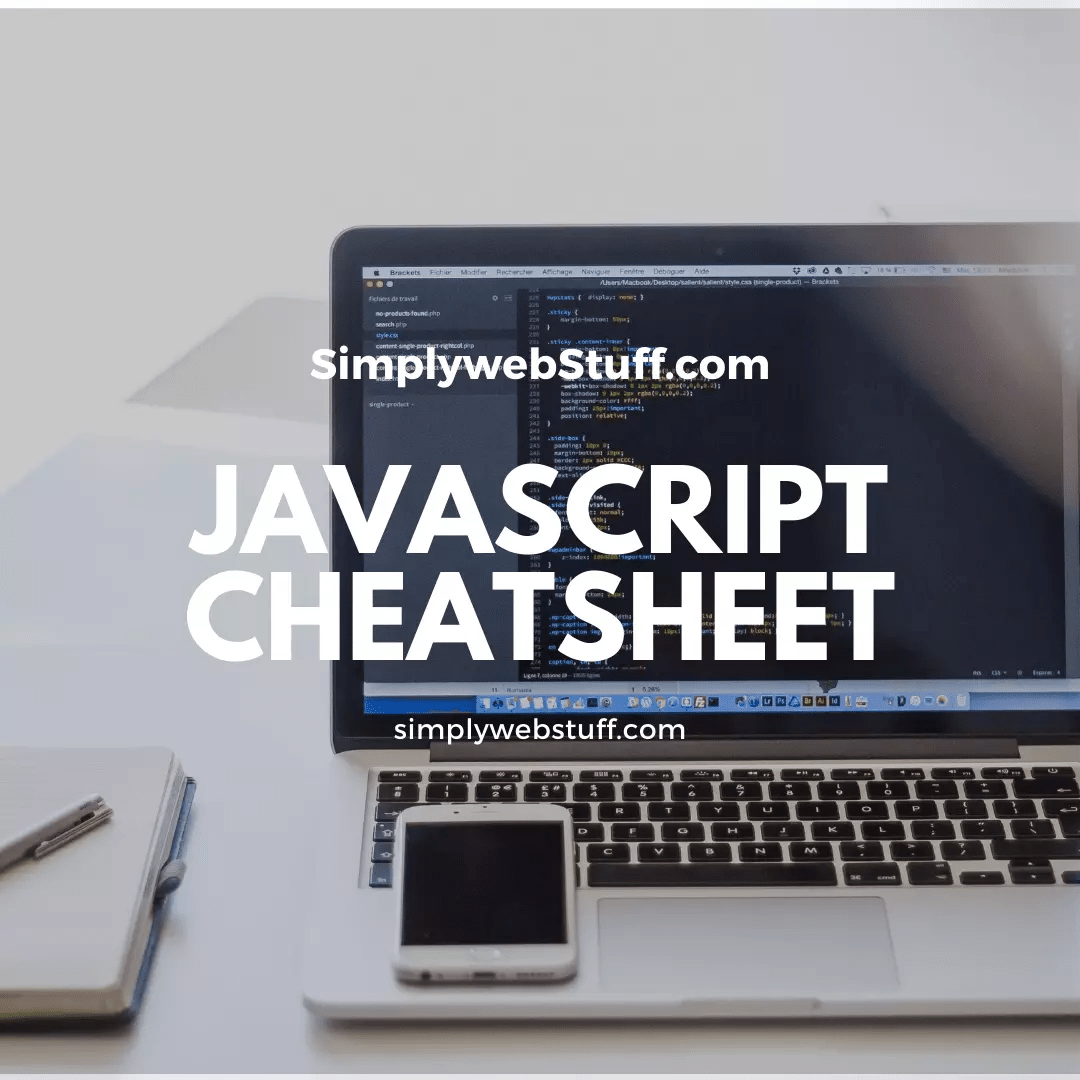
As you may know, JavaScript is a programming language that powers the dynamic behavior on most websites. Alongside HTML and CSS, it is a core technology that runs the web. In this article, I’ll give you a JavaScript cheat sheet that will help you in your JavaScript journey.
In this article you will find:
- JavaScript Array Functions
- JavaScript Boolean Methods
- JavaScript Arithmetic Operators
- JavaScript Global Functions
- JavaScript Loops
- JavaScript Logical Operators
- JavaScript Bitwise Operators
- JavaScript Functions
- JavaScript Escape Characters
- JavaScript String Methods
- JavaScript Pattern Modifiers
- Brackets
- Metacharacters
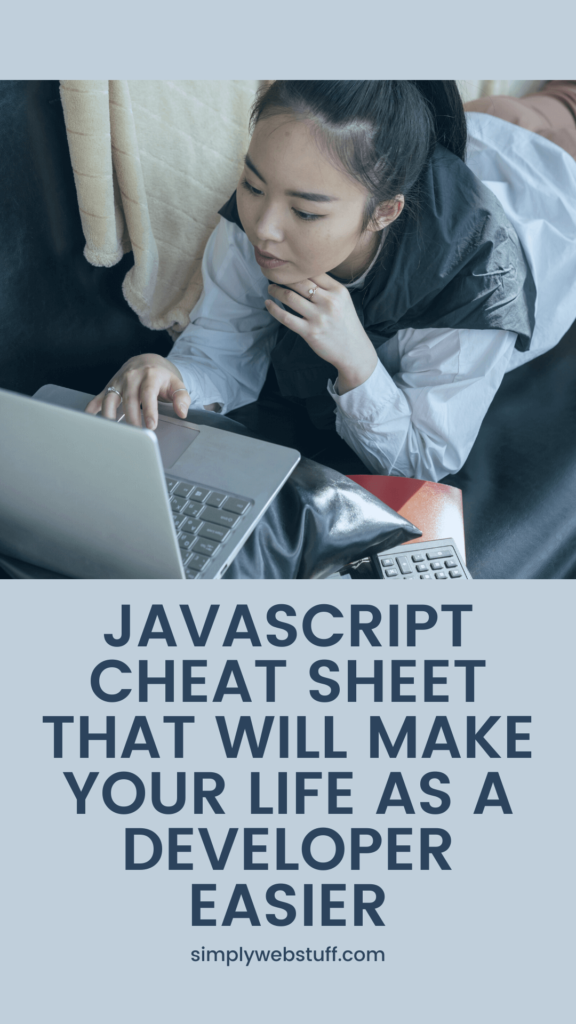
JavaScript Array Functions
- concat() -> Join several arrays into one
- copyWithin() ->Copy array elements within the array, to and from specified positions
- indexOf() -> Return the primitive value of the specified object
- includes() -> Check if an array contains the specified element
- join() -> Combine elements of an array into a single string and return the string
- entries() -> Return a key/value pair Array iteration Object
- every() ->Check if every element in an array with a static value
- fill() -> Fill the elements in an array with a static value
- filter() -> Create a new array with every element in an array that passes a test
- find() -> Return the value of the first element in an array that passes a test
- forEach() -> Call a function for each array element
- from() -> Create an array from an object
- lastIndexOF() -> Give the last position at which a given element appears in an array
- pop() -> Remove the last element of an array
- push() -> Add a new element at the end of an array
- reverse() -> Sort elements in descending order
- reduce() -> Reduce the values of an array to a single value (going left to right)
- reduceRight() -> Reduce the values of an array to a single value(going right to left)
- shift() -> Remove the first element of an array
- slice() -> Pull a copy of a portion of an array into a new array object
- sort() -> Sort elements alphabetically
- splice() -> Add elements in a specified way and position
- unshift() -> Add a new element to the beginning of an array
JavaScript Boolean Methods
- toString() -> Convert a Boolean value to a string and return the result
- valueOf() -> Return the first position at which a given element appears in an array
- toSource() -> Return a string representing the source code of the object
JavaScript Arithmetic Operators
- + -> Addition
- – -> Substraction
- * -> Multiplication
- / -> Division
- (…) -> Grouping operator (operations within brackets are executed earlier than those outside)
- % -> Modulus (remainder)
- ++ -> Increment numbers
- — -> Decrement numbers
- == -> Equal to
- === -> Equal value and equal type
- != -> Not Equal
- !== -> Not equal value or not equal type
- > -> Greater than
- < -> Lesser than
- >= -> Greater than or equal to
- <= -> Lesser than or equal to
- ? -> Ternary operator
JavaScript Global Functions
- decodeURI() -> Decode a Uniform Resource Identifier (URI) created by encode URI
- decodeURIComponent() -> Decode a URI component
- encodeURI() -> Encode a URI into UTF-8
- encodeURIComponent() -> Same but for URI components
- eval() -> Evaluate JavaScript code represented as a string
- inFinite() -> Determine whether a value is a finite number
- isNan() -> Determine whether a value is a number
- Number() -> Convert an object’s value to a number
- parseFloat() -> Parse a string and return a floating point number
- parseInt() -Parse a string and return an integer
JavaScript Loops
- for -> The most common way to create a loop in JavaScript
- while -> Set up conditions under which a loop executes
- do while -> Similar to the while loop but it executes at least once and performs a check at the end to see if the condition is met to execute again
- break -> Stop and exit the cycle if certain conditions are met
- continue -> Skip parts of the cycle if certain conditions are met
JavaScript Logical Operators
- && -> Logical AND
- || -> Logical OR
- ! -> Logical NOT
JavaScript Bitwise Operators
- & -> AND statement
- | -> OR statement
- ^ -> XOR
- ~ -> NOT
- << -> Left shift
- >> -> Right shift
- > -> Shift
JavaScript Functions
- alert() -> Output data in an alert box in the browser’s window
- confirm() -> Open up yes/no dialog and return true/false result
- console.log() -> Write information to the browser’s console
- document.write() -> Write directly to the HTML document
- prompt() -> Create a dialog for user input
JavaScript Escape Characters
- \’ -> Single quote
- \” -> Double quote
- \\ -> Bachslash
- \b -> Backspace
- \f -> Form feed
- \n -> New line
- \r -> Carriage return
- \t -> Horizontal tabulator
- \v -> Vertical tabulator
JavaScript String Methods
- charAt() -> Return character at a specified position inside a string
- charCodeAt() -> Give the Unicode of the character at that position
- concat() -> Concatenate two or more strings into one
- fromCharCode() -> Return a string created from the specified sequence of UTF-16 code units
- indexOf() -> Provide the position of the first occurrence of specified text within a string
- lastIndexOf() -> Same as indexOf() but returns last occurrence
- match() -> Retrieve the matches of a string against a search pattern
- replace() -> Find and replace specified text in a string
- search() -> Execute a search for a matching text and return its position
- slice() -> Extract a section of a string and return it as a new string
- split() -> Split a string object into an array of strings at a specified position
- startsWith() -> Check whether a string begins with specified characters
- substr() -> Similar to slice() but extracts a substring depended on a specified number of characters
- substring() -> Similar to slice() but can’t accept negative indices
- toLowerCase() -> Convert string to lowercase
- toUpperCase() -> Convert string to upper case
- valueOf() -> Return the primitive value of a string object
JavaScript Pattern Modifiers
- e -> Evaluate replacement
- i -> Perform case-insensitive matching
- g -> Perform global matching
- m -> Perform multiple line matching
- s -> Treat strings as a single line
- x -> Allow comments and whitespace in pattern
- U -> Ungreedy pattern
JavaScript Brackets
- [abc] -> Find any of the characters in the brackets
- [^abc] -> Find any character not in the brackets
- [0-9] -> Find the digit specified in the brackets
- [A-z] -> Find any character from uppercase A to lowercase z
- (a|b|c) -> Find any of the alternatives separated with |
JavaScript Metacharacters
- . -> Find a single character, except newline or line terminator
- \w -> Word character
- \W -> Non-word character
- \d -> A digit
- \D -> A non-digit character
- \s -> Whitespace character
- \S -> Non-whitespace character
- \b -> Find a match at the beginning/end of a word
- \B -> Find a match not at the beginning/end of a word
- \u0000 -> NUL character
- \n -> A new line character
- \f -> Form feed character
- \r -> Carriage return character
- \t -> Tab character
- \v -> Vertical tab character
- \xxx -> Character specified by an octal number xxx
- \xxd -> Latin character specified by a hexadecimal number dd
- \udddd -> Unicode character specified by a hexadecimal number dddd