What Are JavaScript Events And How To Use JavaScript Events
Posted by TotalDC
JavaScript events provide a dynamic interface to a webpage. These events are hooked to elements in the DOM (Document Object Model). These events by default use bubbling propagation, upwards in the DOM from children to parent. You can bind events either as inline or in an external script.
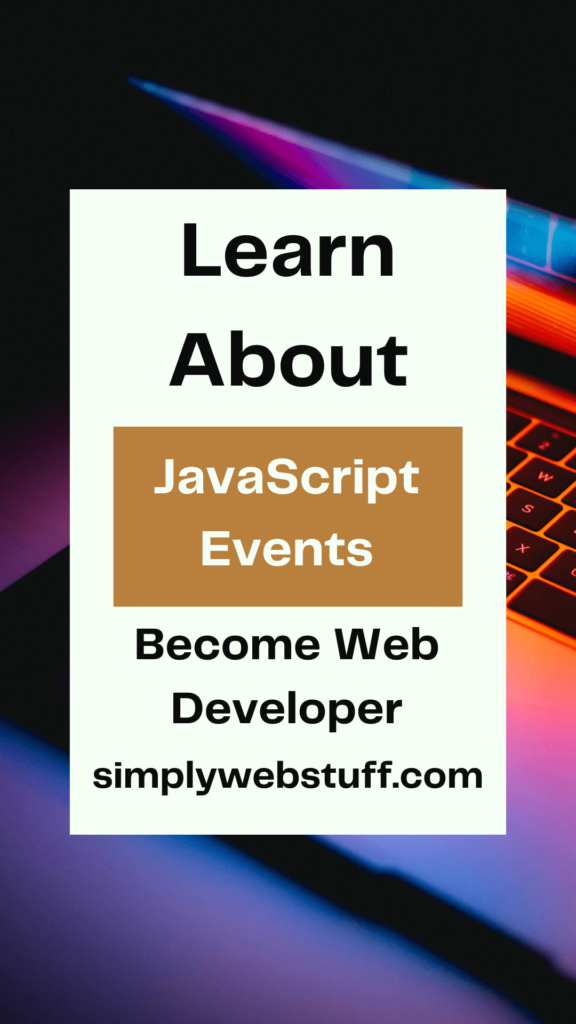
Let’s look at some JavaScript events.
- onclick events: This is a mouse event and provokes any logic defined if the user clicks on the element it is bound to.
<html>
<head>
<script>
function hi() {
alert('Hi there!');
}
</script>
</head>
<body>
<button type="button" onclick="hi()">Click here</button>
</body>
</html>
If you copy this code and open it in your browser you will see a button with “Click here” text on it and when clicked, an alert pop-up will appear with “Hi there” text.
2. onkeyup events: This is a keyboard event that executes instructions whenever a key is released after pressing.
<head>
<script>
var a = 0;
var b = 0;
var c = 0;
function changeBackground() {
var x = document.getElementById('bg');
bg.style.backgroundColor = 'rgb('+a+', '+b+', '+c+')';
a += 1;
b += a + 1;
c += b + 1;
if (a > 255) a = a - b;
if (b > 255) b = a;
if (c > 255) c = b;
}
</script>
</head>
<body>
<input id="bg" onkeyup="changeBackground()"
placeholder="write something" style="color:#fff">
</body>
3. onmouseover event: This event corresponds to hovering the mouse pointer over the element.
<head>
<script>
function hov() {
var e = document.getElementById('hover');
e.style.display = 'none';
}
</script>
</head>
<body>
<div id="hover" onmouseover="hov()"
style="background-color:green;height:200px;width:200px;">
</div>
</body>
Basically what happens here is that when you hover your cursor over the green square then the CSS element of that square gets another attribute “display: none” and disappears from your screen.
4. onmouseout event: Whenever the mouse cursor leaves the element that handles a mouseout event a function is called.
<head>
<script>
function out() {
var e = document.getElementById('hover');
e.style.display = 'none';
}
</script>
</head>
<body>
<div id="hover" onmouseout="out()"
style="background-color:green;height:200px;width:200px;">
</div>
</body>
Same idea as in the previous example but now the square disappears when you take the cursor away from the green square.
5. onchange event: This event detects the change in the value of any element listing to this event.
<head></head>
<body>
<input onchange="alert(this.value)" type="number">
</body>
6. onload event: When an element is loaded completely this event is called.
<head></head>
<body>
<img onload="alert('Image completely loaded')"
alt="GFG-Logo"
src="logo.png">
</body>
When you open this page in a browser and the “logo.png” image is loaded you will get a pop-up message stating that the image has loaded completely.
7. onfocus event: An element listing to this event executes instructions whenever it receives focus.
<head>
<script>
function focused() {
var e = document.getElementById('inp');
if (confirm('Got it?')) {
e.blur();
}
}
</script>
</head>
<body>
<p >Take the focus into the input box below:</p>
<input id="inp" onfocus="focused()">
</body>
In this example to call a function you have to click inside the input field and then you’ll get the alert pop-up message.
8. onblur event: This event is evoked when an element loses focus.
<head></head>
<body>
<p>Write something in the input box and then click elsewhere
in the document body.</p>
<input onblur="alert(this.value)">
</body>
Very similar to the onfocus event but now you have to click inside the input field, write something, and then click anywhere outside of the input field. Then you will get a pop-up with whatever text you have entered.