How To Upload File In PHP
Posted by TotalDC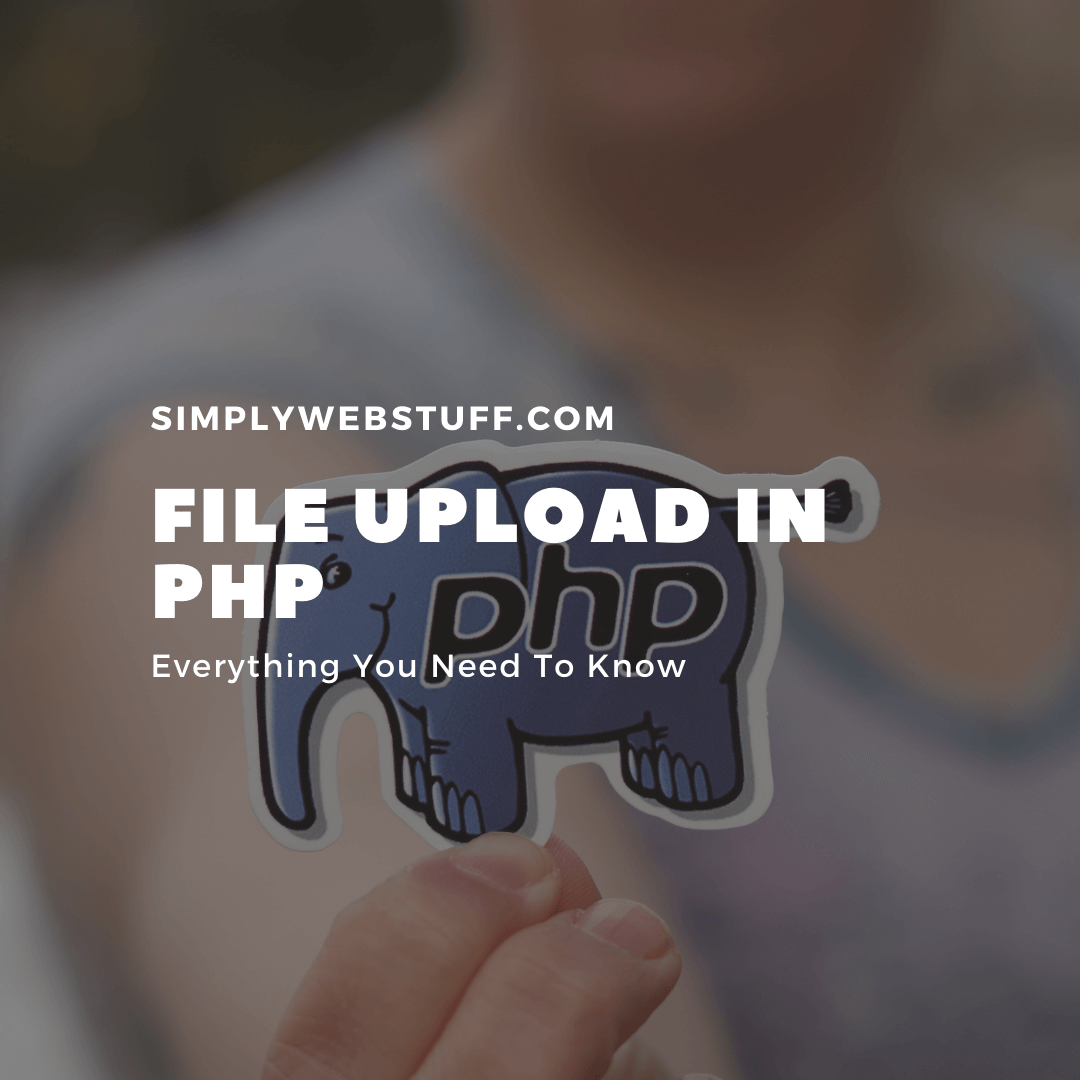
In this tutorial, we will continue our PHP series and learn how to upload files in PHP to a remote server using a simple HTML form.
Uploading Files In PHP
You can upload any kind of file for example images, videos, ZIP files, MS Office documents, PDFs etc.
How To Create HTML Form To Upload The File
Here is an example of how you can create a simple HTML form that can be used to upload files:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>File Upload Form</title>
</head>
<body>
<form action="upload-manager.php" method="post" enctype="multipart/form-data">
<h2>File Upload</h2>
<label for="fileSelect">Filename:</label>
<input type="file" name="photo" id="fileSelect">
<input type="submit" name="submit" value="upload">
<p><strong>Note:</strong> Only .jpg, .jpeg, .gif, .png formats allowed. Max size of 5 MB.</p>
</form>
</body>
</html>
How To Process The Uploaded File In PHP
Now let’s look at the code of the “upload-manager.php” file. It stores the uploaded file in an upload folder permanently as well as implements some basic security checks like file type and file size to ensure that users upload the correct file type and that the file is within the size limit.
<?php
// Check if the form was submitted
if($_SERVER["REQUEST_METHOD"] == "POST"){
// Check if file was uploaded without errors
if(isset($_FILES["photo"]) && $_FILES["photo"]["error"] == 0){
$allowed = array("jpg" => "image/jpg", "jpeg" => "image/jpeg", "gif" => "image/gif", "png" => "image/png");
$filename = $_FILES["photo"]["name"];
$filetype = $_FILES["photo"]["type"];
$filesize = $_FILES["photo"]["size"];
// Verify file extension
$ext = pathinfo($filename, PATHINFO_EXTENSION);
if(!array_key_exists($ext, $allowed)) die("Error: Please select a valid file format.");
// Verify file size - 5MB maximum
$maxsize = 5 * 1024 * 1024;
if($filesize > $maxsize) die("Error: File size is larger than the allowed limit.");
// Verify MYME type of the file
if(in_array($filetype, $allowed)){
// Check whether file exists before uploading it
if(file_exists("upload/" . $filename)){
print $filename . " is already exists.";
} else{
move_uploaded_file($_FILES["photo"]["tmp_name"], "upload/" . $filename);
print "Your file was uploaded successfully.";
}
} else{
print "Error: There was a problem uploading your file. Please try again.";
}
} else{
print "Error: " . $_FILES["photo"]["error"];
}
}
?>
Now let’s look at each part of this example code one by one for a better understanding of this process.
File Upload PHP Code Explanation
When the form is submitted, information about the uploaded file can be accessed via PHP superglobal array $_FILES. For example, your upload form contains a file select field called photo (name=”photo”), if the user uploads a file using this field, you can obtain its details like name, type, size, temporary name, or any error that occurred while attempting to upload. You can do that by using $_FILES[“photo”] associative array, like this:
$_FILES["photo"]["name"]
— This array value specifies the original name of the file, including the file extension. It doesn’t include the file path.$_FILES["photo"]["type"]
— This array value specifies the MIME type of the file.$_FILES["photo"]["size"]
— This array value specifies the file size, in bytes.$_FILES["photo"]["tmp_name"]
— This array value specifies the temporary name including the full path that is assigned to the file once it has been uploaded to the server.$_FILES["photo"]["error"]
— This array value specifies the error or status code associated with the file upload, e.g. it will be 0 if there is no error.
The PHP code in the next example will simply display the details of the uploaded file and store it in a temporary directory on the web server.
<?php
if($_FILES["photo"]["error"] > 0){
print "Error: " . $_FILES["photo"]["error"] . "<br>";
} else{
print "File Name: " . $_FILES["photo"]["name"] . "<br>";
print "File Type: " . $_FILES["photo"]["type"] . "<br>";
print "File Size: " . ($_FILES["photo"]["size"] / 1024) . " KB<br>";
print "Stored in: " . $_FILES["photo"]["tmp_name"];
}
?>
When the file has been successfully uploaded, it is automatically stored in a temporary directory on the server. To store this file permanently, you need to move it from the temporary directory to a permanent location using the PHP’s move_uploaded_file() function.