Everything You Need To Know About Session In PHP
Posted by TotalDC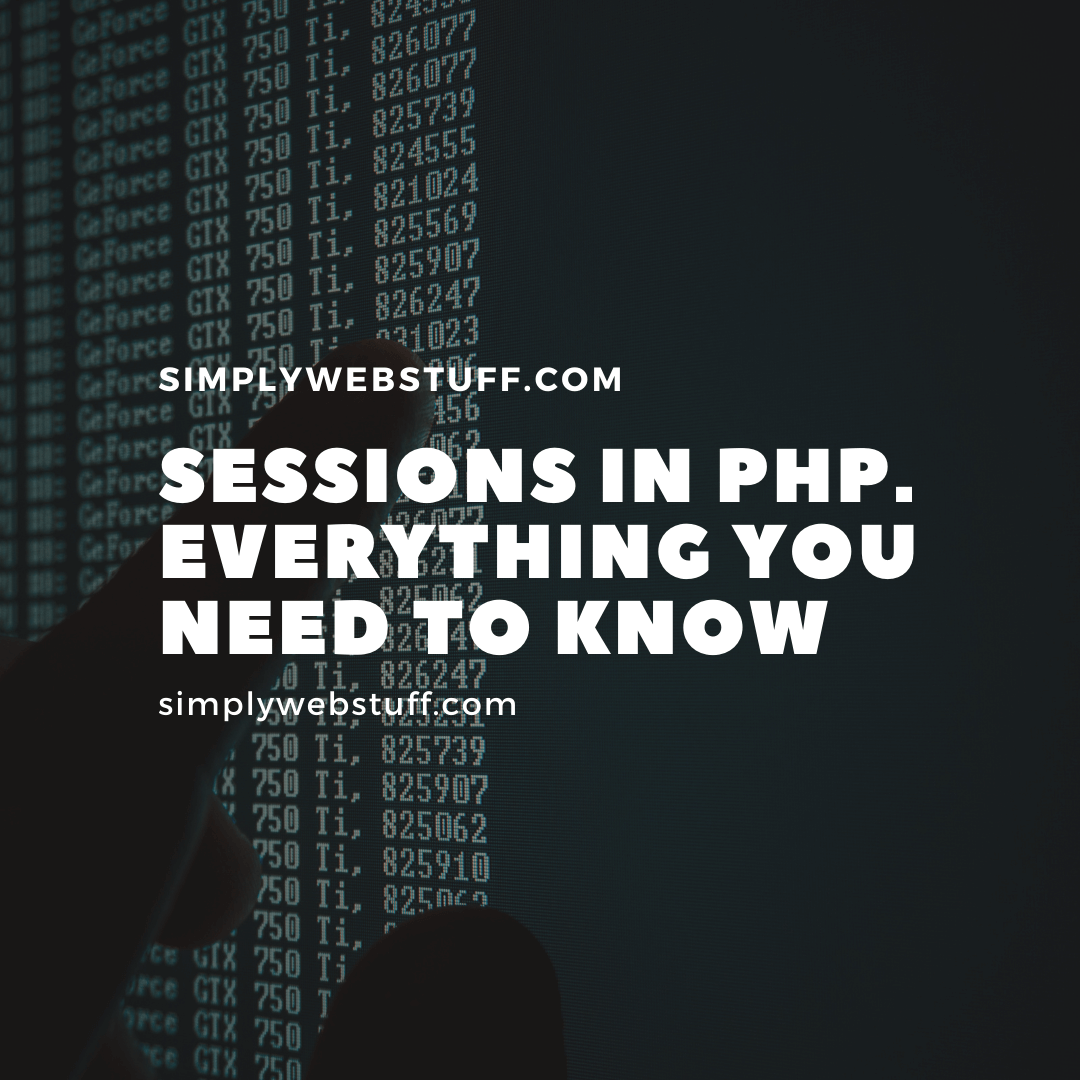
Not that you learn what are cookies, how to create them, and how to delete them, but it’s time to move even further and talk about sessions. In this article, you will learn what sessions are, how to create, and how to destroy a session in PHP.
What Is Session In PHP
If you read the last post on this blog, you know that you can store data using cookies. However, it has some security problems since cookies are stored on the user’s computer. It is possible to easily modify cookie content to insert potentially malicious data that might break your application.
Also, cookies can affect your website’s performance because every time the browser sends a request to the server, all the cookie data for a website is sent. It means that if you have stored 5 cookies 4KB in size on the user’s computer, the browser needs to upload 20KB of data each time the user views a page.
But you can solve both of these issues by using a PHP session. A PHP session stores data on the server rather than the user’s computer. In a session-based environment, every user is identified through a unique number called a session identifier (SID). This unique session ID is used to link each user with their information on the server. For example emails, pots, etc.
How To Start a PHP Session
To store any information in the session, you must first start up the session. To begin a new session, simply call the PHP session_start() function. It will create a new session and generate a unique ID for the user.
Here is an example of how to start a new session:
<?php
session_start();
?>
The session_start() function checks if a session already exists by looking for the session ID. If the session is already started, it sets up the session variables and if it doesn’t, it starts a new session by creating a new session ID.
How To Store And Access Session Data
You can store all your session data as key-value pairs in the $_SESSION[] superglobal array. The stored data can be accessed during the lifetime of a session. Here’s the script which creates a new session and registers two session variables.
<?php
session_start();
$_SESSION["firstname"] = "Web";
$_SESSION["lastname"] = "Stuff";
?>
Now here is an example of how to access data that you have stored in session:
<?php
echo 'Hi, ' . $_SESSION["firstname"] . ' ' . $_SESSION["lastname"];
?>
Your result would be:
Web Stuff
How To Destroy A Session In PHP
Let’s say you want to remove certain session data. In that case, simply unset the corresponding key of the $_SESSION associative array:
<?php
if(isset($_SESSION["lastname"])){
unset($_SESSION["lastname"]);
}
?>
And if you want to destroy a session completely, call the session_destroy() function. This function does not need any argument and a single call destroys all the session data.
<?php
session_destroy();
?>
Worth noticing that every PHP session has a timeout value or duration which is measured in seconds. You can adjust this timeout duration by changing the value of session.gc_maxlife variable in the PHP configuration in the php.ini file.