What To Know About PHP Date And Time
Posted by TotalDC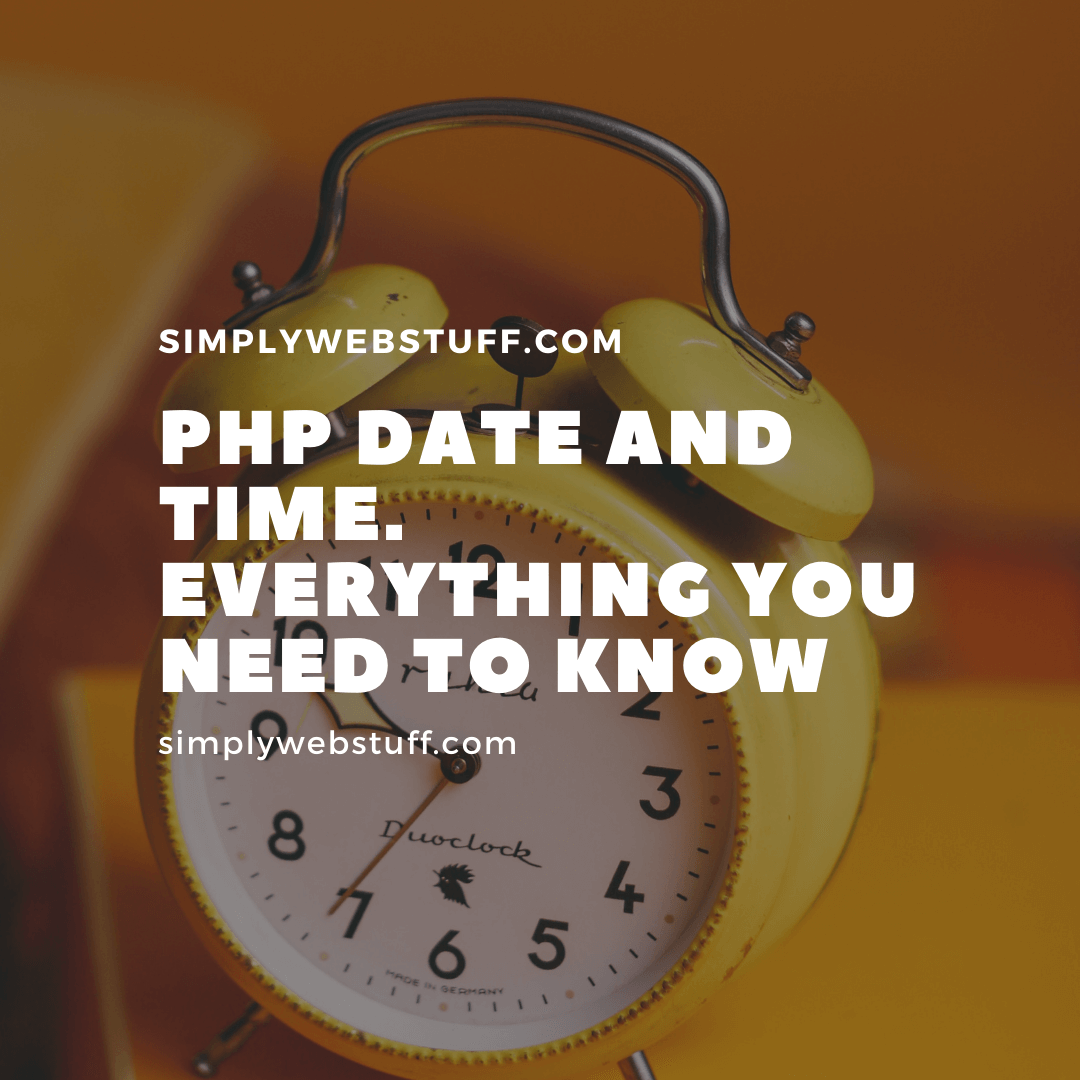
Last time we talked about GET and POST methods. Now let’s continue our PHP series by talking about PHP date and time. Here you will learn how to use PHP date and time functions.
The PHP Date() Function
The PHP date() function converts a timestamp to an understandable date and time.
Originally computers store date and time in the UNIX Timestamp which measures time as seconds since the midnight Greenwich Mean Time on January 1, 1970 (1970 00:00:00 GMT) or in other words the beginning of the Unix epoch.
As you can see this is an impractical format for us to read. PHP converts a timestamp to a format that is readable to humans and dates from your notation into a timestamp the computer understands. The syntax of the PHP date() function looks like this:
date(format, timestamp)
The format parameter in the date() function is required which specifies the format of returned date and time. But then timestamp is an optional parameter. If you don’t include it – the current date and time will be used. Let’s look at the example of the function displaying today’s date:
<?php
$today = date("d/m/Y");
print $today;
?>
Formatting Date And Time With PHP
The format parameter of the date() function can contain multiple characters allowing you to generate a string containing various components of the date and time, for example, day of the week, AM, PM, and more. Here are commonly used date formatting characters:
- d – Represent the day of the month; two digits with leading zeros (01 or 31)
- D – Represent the day of the week in text as an abbreviation (Mon to Sun)
- m – Represent month in numbers with leading zeros (01 or 12)
- M – Represent month in text, abbreviated (Jan to Dec)
- y – Represent the year in two digits (08 or 14)
- Y – Represent the year in four digits (2019 or 2021)
The parts of the date can be separated by inserting characters like hyphens (-), dots (.), slashes(/), or spaces to add visual formatting. Here is an example:
<?php
print date("d/m/Y") . "<br>";
print date("d-m-Y") . "<br>";
print date("d.m.Y");
?>
Here is a list of characters you can use to format the time string:
- h – Represent hour in 12-hour format with leading zeros (01 to 12)
- H – Represent hour in 24-hour format with leading zeros (00 to 23)
- i – Represent minutes with leading zeros (00 to 59)
- s – Represent seconds with leading zeros (00 to 59)
- a – Represent lowercase ante meridiem and post meridiem (am or pm)
- A – Represent uppercase Ante meridiem and Post meridiem (AM or PM)
Here is an example of different time formatting:
<?php
print date("h:i:s") . "<br>";
print date("F d, Y h:i:s A") . "<br>";
print date("h:i a");
?>
Time() Function In PHP
The time() function is used to get the current time as a Unix timestamp (the number of seconds since the beginning of the Unix epoch: January 1 1970 00:00:00 GMT). Example:
<?php
$timestamp = time();
print($timestamp);
?>
Result would be:
1639860765
Now we can convert this timestamp to a readable date by passing it the date() function.
<?php
$timestamp = 1639860765;
print(date("F d, Y h:i:s", $timestamp));
?>
Result would be:
Sat 18 2021 20:52:45
Mktime() Function in PHP
The mktime() function works opposite to date() function and is used to create timestamps based on a specific date and time. And if no date and time are given, you will get a timestamp for the current date and time.
The syntax of the mktime() function looks like this:
mktime(hour, minute, second, month, day, year)
The following example displays the timestamp corresponding to 3:20:12 pm on December 18, 2021:
<?php
print mktime(15, 20, 12, 12, 18, 2014);
?>
The mktime() function can be used to find the weekday name of a particular date. To do this you can simply use the ‘I’ (lowercase L) character with your timestamp.
<?php
print date('l', mktime(0, 0, 0, 4, 1, 2021));
?>
The result of that is:
Thursday
This function can also be used to find dates after a specific time period. As in the following example date after 5 months from now:
<?php
$futureDate = mktime(0, 0, 0, date("m")+5, date("d"), date("Y"));
print date("d/m/Y", $futureDate);
?>
Result:
18/05/2022