What You Need To Know About JSON Parsing In PHP
Posted by TotalDC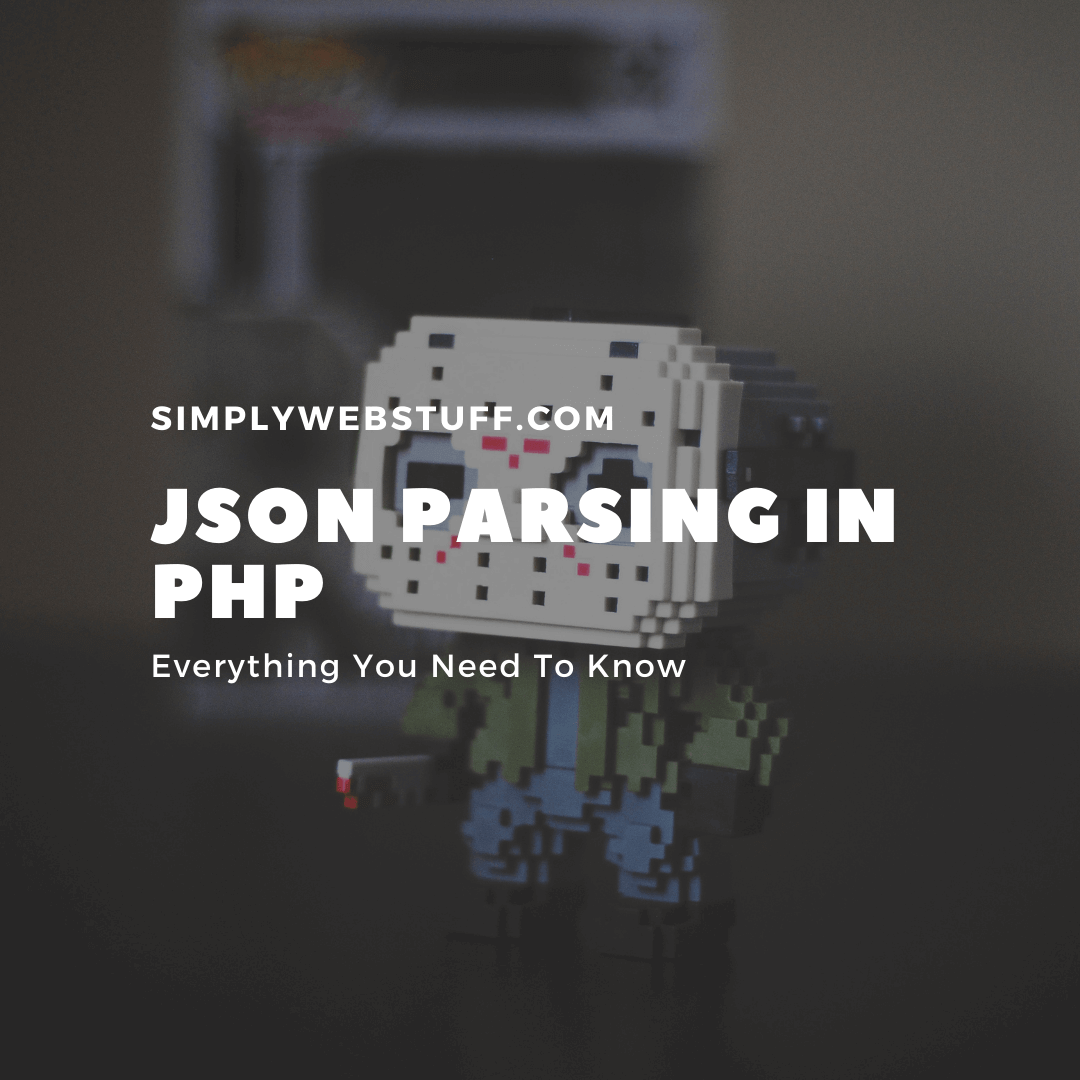
Last time we covered classes and objects in PHP, now let’s look at JSON. In this tutorial, you will learn everything you need to know about JSON parsing in PHP.
What Is JSON In PHP
Perhaps you’ve seen the article on JSON from a JavaScript point of view, then you know what JSON is, but if not here’s a short introduction.
JSON – JavaScript Object Notation. JSON is a standard data interchange format that is quick and easy to create and parse.
JSON is a text-based format that is easy to understand both for us humans and for computers. JSON is based on two basic structures:
- Object – This is defined as a collection of key/value pairs. Each object begins with a left curly bracket. Multiple key/value pairs are separated by a comma.
- Array – This is defined as an ordered list of values. An array begins with a left bracket. Values are separated by a comma.
In JSON, keys are always strings, but the value can be a string, number, boolean, null, object, or array. Strings must be enclosed in double quotes and can contain escape characters. For example, simple JSON object could look like this:
{
"website": {
"name": "simplywebstuff.com",
"author": "TotalDC",
"year": 2021,
"genre": "Web Stuff"
}
}
And simple JSON array could look like this:
{
"languages": [
"javascript",
"php",
"python",
"java"
]
}
How To Parse JSON In PHP
As you can see, data structures are similar to PHP arrays. And PHP has built-in functions json_encode() and json_decode(), which are used to encode and decode JSON files. Both functions only work with UTH-8 encoded data.
How To Encode JSON Data In PHP
PHP uses the json_encode() function to encode data in JSON format. Here is an example that demonstrates how to encode a PHP associative array into a JSON object:
<?php
// An associative array
$person = array("Peter"=>65, "Harry"=>80, "John"=>78, "Clark"=>90);
print json_encode($person);
?>
The output of the above example will look like this:
{"Peter":65,"Harry":80,"John":78,"Clark":90}
Also you can encode PHP indexed array. Here’s an example:
<?php
// An indexed array
$colors = array("Red", "Green", "Blue", "Orange", "Yellow");
print json_encode($colors);
?>
The output of the above example will look like this:
["Red","Green","Blue","Orange","Yellow"]
You can even force json_encode() function to return a PHP-indexed array as a JSON object by using JSON_FORCE_OBJECT. Let’s look at the example:
<?php
// An indexed array
$colors = array("Red", "Green", "Blue", "Orange");
print json_encode($colors, JSON_FORCE_OBJECT);
?>
The output of the above example will look like this:
{"0":"Red","1":"Green","2":"Blue","3":"Orange"}
As you can see in the above examples a non-associative array can be encoded as array or object. However, an associative array is always encoded as an object.
How To Decode JSON In PHP
Well, decoding JSON is as simple as encoding it. You can use the json_decode() function for that. The following example demonstrates how to decode a JSON object to a PHP object.
<?php
// Store JSON data in a PHP variable
$json = '{"Peter":65,"Harry":80,"John":78,"Clark":90}';
var_dump(json_decode($json));
?>
The result of the example will look like this:
object(stdClass)#1 (4) { ["Peter"]=> int(65) ["Harry"]=> int(80) ["John"]=> int(78) ["Clark"]=> int(90) }
By default, the json_decode() function returns an object. But you can specify a second parameter $assoc which accepts a boolean value that if set as true will return an associative array. Let’s look at the example:
<?php
// Store JSON data in a PHP variable
$json = '{"Peter":65,"Harry":80,"John":78,"Clark":90}';
var_dump(json_decode($json, true));
?>
Now the result will look like this:
array(4) { ["Peter"]=> int(65) ["Harry"]=> int(80) ["John"]=> int(78) ["Clark"]=> int(90) }
Here is an example that shows how to decode JSON and access individual elements of the JSON object or array in PHP.
<?php
// Assign JSON encoded string to a PHP variable
$json = '{"Peter":65,"Harry":80,"John":78,"Clark":90}';
// Decode JSON data to PHP associative array
$arr = json_decode($json, true);
// Access values from the associative array
print $arr["Peter"]; // Output: 65
print $arr["Harry"]; // Output: 80
print $arr["John"]; // Output: 78
print $arr["Clark"]; // Output: 90
// Decode JSON data to PHP object
$obj = json_decode($json);
// Access values from the returned object
print $obj->Peter; // Output: 65
print $obj->Harry; // Output: 80
print $obj->John; // Output: 78
print $obj->Clark; // Output: 90
?>
I hear you saying why are you not using foreach() look? Here is an example with foreach():
<?php
// Assign JSON encoded string to a PHP variable
$json = '{"Peter":65,"Harry":80,"John":78,"Clark":90}';
// Decode JSON data to PHP associative array
$arr = json_decode($json, true);
// Loop through the associative array
foreach($arr as $key=>$value){
print $key . "=>" . $value . "<br>";
}
echo "<hr>";
// Decode JSON data to PHP object
$obj = json_decode($json);
// Loop through the object
foreach($obj as $key=>$value){
print $key . "=>" . $value . "<br>";
}
?>
How To Extract Values From Nested JSON In PHP
JSON objects and arrays can also be nested. JSON objects can contain other JSON objects, arrays, nested arrays, arrays of JSON objects, etc. This example will show you how to decode a nested JSON object in PHP:
<?php
// Define recursive function to extract nested values
function printValues($arr) {
global $count;
global $values;
// Check input is an array
if(!is_array($arr)){
die("ERROR: Input is not an array");
}
foreach($arr as $key=>$value){
if(is_array($value)){
printValues($value);
} else{
$values[] = $value;
$count++;
}
}
// Return total count and values found in array
return array('total' => $count, 'values' => $values);
}
// Assign JSON encoded string to a PHP variable
$json = '{
"blog": {
"name": "simplywebstuff.com",
"author": "TotalDC",
"year": 2021,
"languages": ["JavaScript", "PHP", "CSS"],
"genre": "WebDev",
"price": {
"subscription": "free"
}
}
}';
// Decode JSON data into PHP associative array format
$arr = json_decode($json, true);
// Call the function and print all the values
$result = printValues($arr);
print "<h3>" . $result["total"] . " value(s) found: </h3>";
print implode("<br>", $result["values"]);
print "<hr>";
// Print a single value
print $arr["blog"]["author"] . "<br>"; // Result: TotalDC
print $arr["blog"]["languages"][0] . "<br>"; // Result: JavaScript
print $arr["blog"]["price"]["subscription"]; // Result: free
?>