Everything You Need To Know About JavaScript Window Object
Posted by TotalDC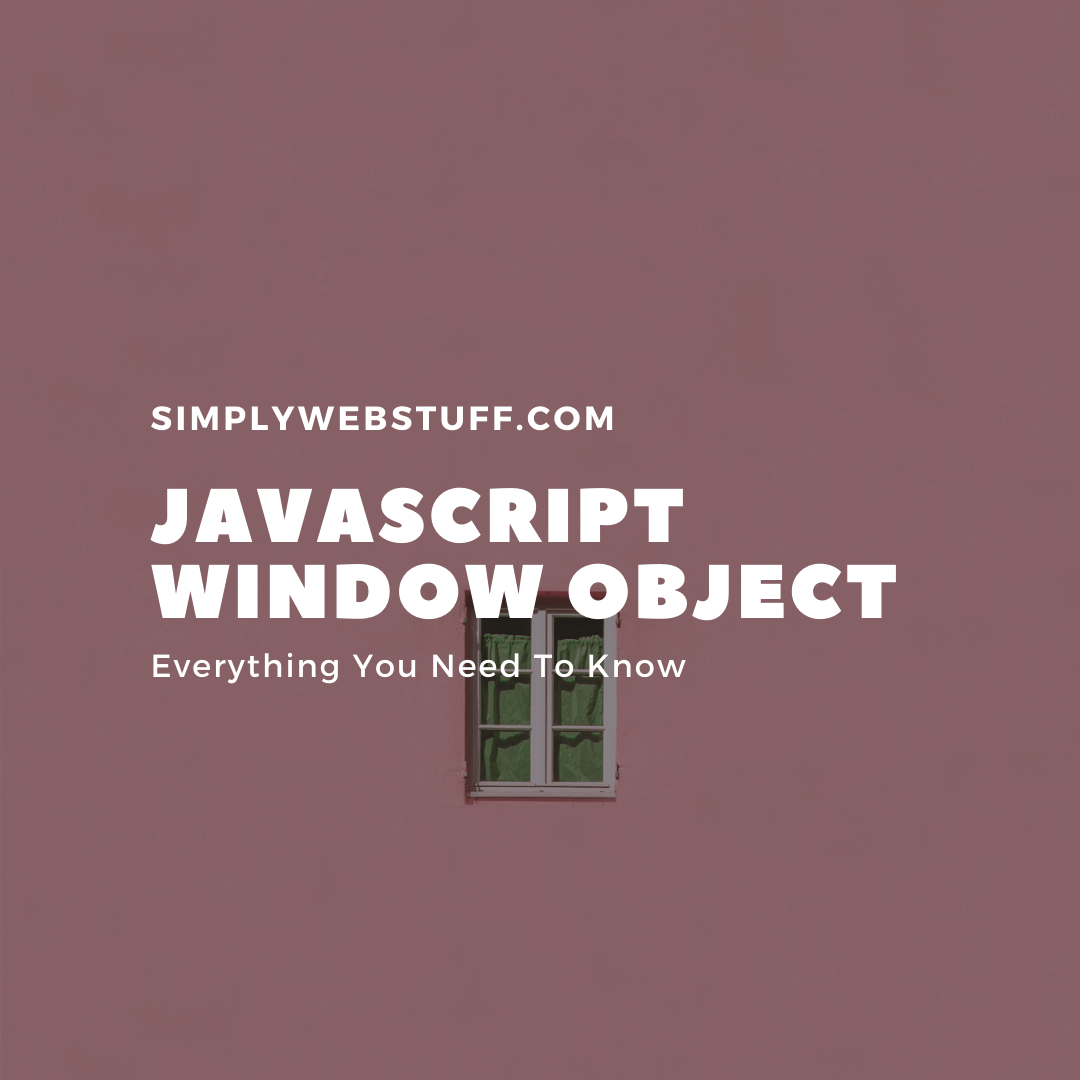
The JavaScript window object basically represents a window containing a DOM document. A window can be the main window, a frame set or individual frame, or even a new window created using JavaScript.
For example alert() method that we used in some previous tutorials to show popup messages is a method of the window object.
How To Calculate Width And Height Of The Window Using JavaScript
The window object provides the innerWidth and innerHeight properties to find out the width and height of the browser window viewport (in pixels) including the horizontal and vertical scrollbar, if rendered. Here’s an example that displays the current size of the window on button click:
<script>
function windowSize(){
let w = window.innerWidth;
let h = window.innerHeight;
alert("Width: " + w + ", " + "Height: " + h);
}
</script>
<button type="button" onclick="windowSize();">Get Window Size</button>
And if you want to find out the width and height of the window excluding the scrollbars you need to use the clientWidth and clientHeight properties of any DOM element. Here’s how it looks:
<script>
function windowSize(){
let w = document.documentElement.clientWidth;
let h = document.documentElement.clientHeight;
alert("Width: " + w + ", " + "Height: " + h);
}
</script>
<button type="button" onclick="windowSize();">Get Window Size</button>