How To Sort Array In JavaScript
Posted by TotalDC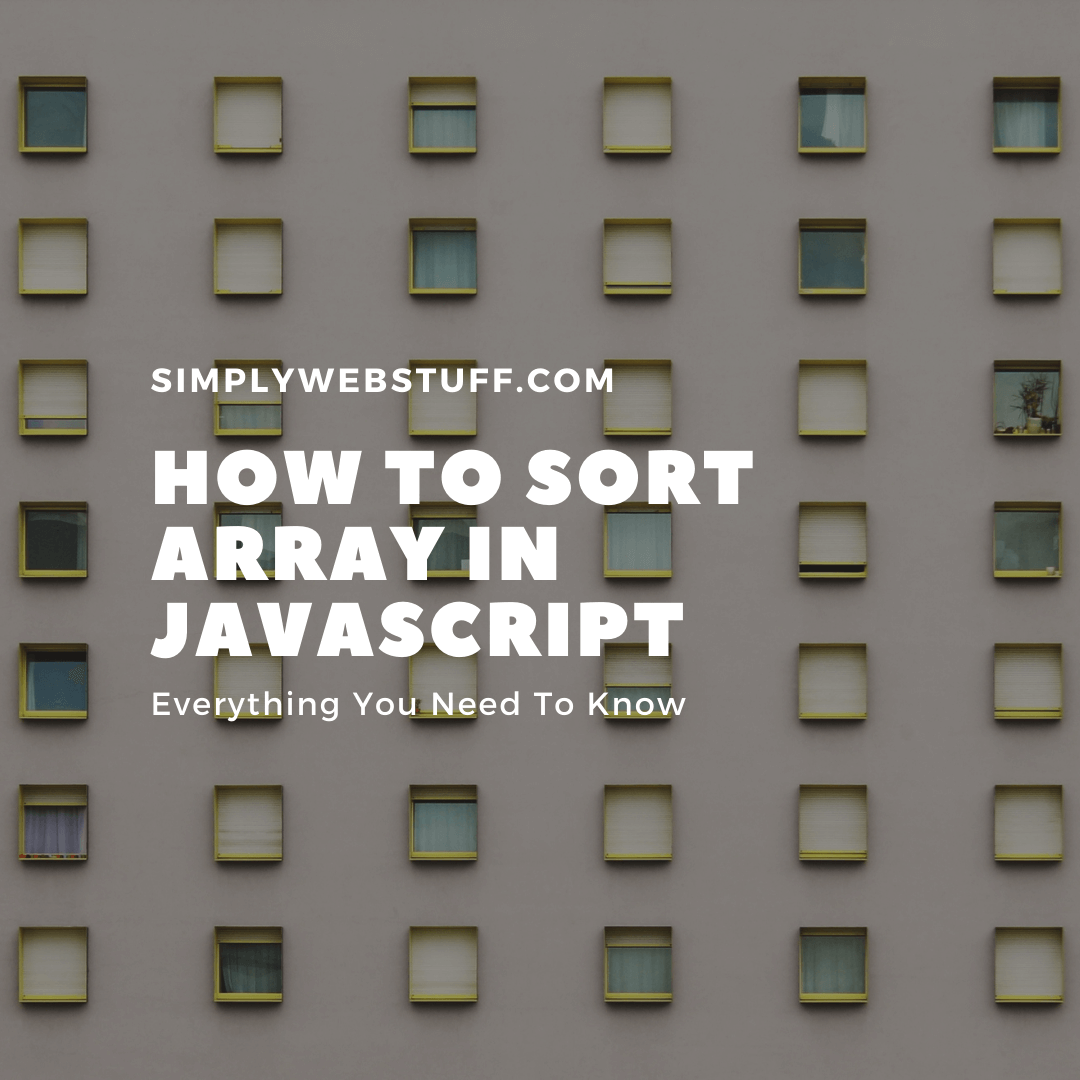
In previous post we looked at what is an array in JavaScript, how to create it, and how to work with it in general. Now let’s look at how to sort arrays in JavaScript.
Sorting An Array In JavaScript
Sorting is a very common task when you are working with arrays. You will use sorting, for example, if you want to display data in alphabetical order.
JavaScript Array object has a built-in method sort() for sorting array elements in alphabetical order. The following example shows how it works:
let fruits = ["Banana", "Orange", "Apple", "Papaya", "Mango"];
let sorted = fruits.sort();
console.log(fruits); // Result: Apple,Banana,Mango,Orange,Papaya
console.log(sorted); // Result: Apple,Banana,Mango,Orange,Papaya
How To Reverse An Array In JavaScript
For this task, you can use the reverse() method to reverse the order of the elements in the array.
As you may guess, this method reverses an array in such a way that the first array element becomes the last array element and the last array element becomes the first. Here’s an example for you:
let counts = ["one", "two", "three", "four", "five"];
let reversed = counts.reverse();
console.log(counts); // Outputs: five,four,three,two,one
console.log(reversed); // Output: five,four,three,two,one
How To Sort Numeric Arrays In Javascript
The sort() method may get you an unexpected result when it is applied on the numeric arrays. As shown here:
let numbers = [5, 20, 10, 75, 50, 100];
numbers.sort(); // Sorts numbers array
console.log(numbers); // Result: 10,100,20,5,50,75
The result is not what you wanted, because the sort() method sorts the numeric array elements by converting them to strings, and since the first character of string “20” comes after the first character of string “100”, that’s why the value 20 is put after the 100.
To overcome this issue you need to pass a compare function. Just like this:
let numbers = [5, 20, 10, 75, 50, 100];
// Sorting an array using compare function
numbers.sort(function(a, b) {
return a - b;
});
console.log(numbers); // Result: 5,10,20,50,75,100
How To Find The Maximum And The Minimum Value In An Array In JavaScript
To do that you can use the apply() method in combination with the Math.max() and Math.min() to find the maximum and minimum value of array elements. Let’s look at the example:
let numbers = [3, -7, 10, 8, 15, 2];
// Defining function to find maximum value
function findMax(array) {
return Math.max.apply(null, array);
}
// Defining function to find minimum value
function findMin(array) {
return Math.min.apply(null, array);
}
console.log(findMax(numbers)); // Result: 15
console.log(findMin(numbers)); // Result: -7
The apply() method provides a convenient way to pass array values as arguments to a function that accepts multiple arguments in an array-like manner, but not an array. So the resulting statement Math.max.apply(null, numbers) in the example is equivalent to the Math.max(3,-7,10,8,15,2).
How To Sort An Array Of Objects In JavaScript
The sort() method can also be used for sorting object arrays using the compare function.
Here is an example of how to sort an array of objects by property values:
let persons = [
{ name: "Harry", age: 25 },
{ name: "Ethan", age: 30 },
{ name: "Peter", age: 21 },
{ name: "Clark", age: 42 },
{ name: "Alice", age: 31 }
];
// Sort by age
persons.sort(function (a, b) {
return a.age - b.age;
});
console.log(persons);
// Sort by name
persons.sort(function(a, b) {
let x = a.name.toLowerCase(); // make everything lower case
let y = b.name.toLowerCase(); // make everything lower case
if(x < y) {
return -1;
}
if(x > y) {
return 1;
}
// names must be equal
return 0;
});
console.log(persons);